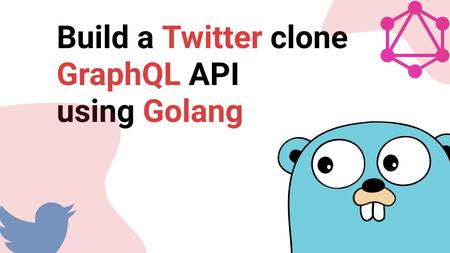
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 34 Lessons (7h 30m) | 1.23 GB
Learn how to use Golang and gqlgen to make a clone of Twitter with a GraphQL API.
Make a GraphQL API using one of the hottest languages!
Golang is quickly becoming one of the most appreciated backend languages. With it’s strong standard library, you can go really far without adding dependencies.
In this tutorial we will use gqlgen, a library made for building a GraphQL API.
Why use gqlgen over other libraries?
One reason: everything is typed. You will almost never need to use an empty interface
How does gqlgen work?
By using Schema First design, gqlgen will generate the Go code for you. This way all your resolvers will be typed safely.
So what does that mean?
You create your API using GraphQL Schema definition language with the shape you want. And you let gqlgen do all the heavy lifting.
So what’s left to do then?
You just need to plug your business logic into the resolvers, that’s it.
Libraries we will use
- PGX
- scany as SQLX for PGX alternative
- gqlgen
- Dataloaden
- golang-migrate
- Insomnia
- Chi
- PostgreSQL
- godotenv
- testify
Table of Contents
1 ToolsNeeded
2 Course Structure
3 Folder Structure
4 Go Modules
5 Intro AuthService and Register
6 Coding AuthService Interface and Validation of the RegisterInput
7 Testing AuthService RegisterInput
8 Coding Register and Dealing With the UserRepo Interface
9 Testing Mockery and how to Mock the UserRepo so we can test the Register Method
10 Coding Add the Login Method
11 Testing Add Tests to our Login Logic
12 How to Speed up our Bcrypt Testing
13 Coding Setup PGX and Install Gotdotenv
14 Coding Add Migrations with Golang Migrate
15 Coding UserRepo Implementation
16 Testing Integration Test for the Register Method and Creation of Test Helpers
17 Testing Improving our Faker Package
18 Intro What is gqlgen
19 Coding Adding gqlgen and Basic Setup of AuthResolver
20 Coding Register Login Resolvers
21 Intro How we will work with the Refresh token
22 Coding Implementation of the Refresh AccessToken Creation and Parsing Logic 1
23 Testing Writing Tests for our JWT Package
24 CodingTesting Create Auth Middleware and Play With Context
25 Coding Testing Using Insomnia for GraphQL Request and fix our Mocks
26 Coding Testing Creating TweetService and TweetRepo Interface
27 Coding Testing Create Tweet Migration and Setup Create Tweet Logic
28 Coding Testing Implement TweetService GetByID and All
29 Coding Implement Create Mutation and Tweets Query
30 Coding Testing Implement TweetService Delete and Plug it to GraphQL
31 Coding Adding the User Relationship to the Tweet
32 Coding Adding Dataloader for the Tweet User Relationship
33 Coding Testing Adding Replies to Tweet
34 Coding – Adding Replies to our GQL Layer
Resolve the captcha to access the links!