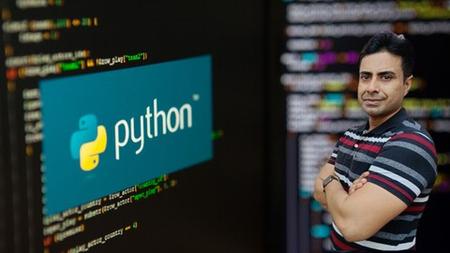
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 134 lectures (7h 52m) | 1.58 GB
Unlock the Power of Python: Learn Installation, Basics, Advanced Techniques, and Dive into Exciting Real-World Projects
Embark on a transformative Python journey with “Python Mastery,” a comprehensive course designed to elevate your programming skills to new heights. Whether you’re a beginner or an intermediate learner, this course provides a seamless progression from foundational concepts to advanced project implementation.
Dive into the intricacies of Python programming, starting with the essentials of installation. Demystify variables and grasp fundamental operators as you lay the groundwork for your Python expertise. Explore the creation and manipulation of powerful collections, delve into modules, and master the art of flow control, gaining a profound understanding of Python’s rich tapestry.
Navigate the Python Shell and learn how to work with modules effectively. Gain insights into file manipulation intricacies, honing your skills in handling CSV files and leveraging the OS module for streamlined directory operations. Immerse yourself in hands-on projects, applying your knowledge to real-world scenarios and conquering challenges with confidence.
Uncover the secrets of functions and unravel the intricacies of Object-Oriented Programming (OOP). Fortify your code with pro-level error handling techniques, ensuring robust and resilient applications. Learn the art of string formatting and discover the power of this essential skill in enhancing the readability and efficiency of your code.
Engage in stimulating projects throughout the course, honing your skills in practical applications. While specific project names are withheld, rest assured that you’ll tackle three immersive and diverse projects, reinforcing your learning and allowing you to apply your knowledge in dynamic scenarios.
This meticulously crafted learning experience goes beyond traditional tutorials, offering a dynamic and engaging approach to mastering Python. With a focus on practicality and real-world applicability, “Python Mastery” equips you with the tools and knowledge needed to navigate Python’s complexities confidently.
Enroll now to join the Python elite, ignite your curiosity, and unleash the full potential of Python programming. Elevate your programming prowess, tackle real-world challenges, and emerge a Python master. Don’t miss out on this opportunity to shape your programming future with “Python Mastery.”
What you’ll learn
- Learn seamless Python installation, setup, and verification for a smooth coding experience.
- Master variables, data types, and string manipulations, laying the groundwork for Python proficiency.
- Develop skills in mathematical, assignment, and comparison operators for effective data manipulation.
- Manipulate lists, tuples, sets, and dictionaries, acquiring versatile skills for diverse data structures.
- Understand Python modules, import them, and explore functionalities using dir() and help() methods.
- Gain expertise in if statements, elif conditions, loops, and conditional statements for efficient program execution.
- Tackle CSV file manipulation, work with the OS module, and create engaging applications in three hands-on projects.
Table of Contents
Introduction
1 Introduction
How To Install Setup Python
2 Get Python on Your Computer
3 Set Up Python on Your System
4 Verify Your Python Installation
5 Using Command Line to Write Python Code
6 ResourceDemopy
7 Using Python py Files
Types and Variables The Building Blocks of Python Programming
8 Understanding Variables Within Python
9 Resource Python Variables
10 Working With Python Numbers
11 Understanding String Methods Within Python
12 Working With Python Strings
13 Reference Material String Methods
14 Casting Data Types Within Python
Python Operators The Essential Tools for Data Manipulation
15 Mathematical Operators In Python
16 Python Assignment Operators
17 Comparison Operators Within Python
18 List Of Commonly Used Python Operators
Working with Python Collections
19 How to Use Lists in Python
20 Creating Lists with the List Constructor
21 Adding Elements to List with the Append Method
22 Exploring Other Useful List Methods
23 Reference Material List Method
24 How to Use Tuples in Python
25 Comparing Lists and Tuples
26 Set Data Within Python
27 Exploring Other Useful Set Methods
28 How to Use Dictionaries in Python
Using Python Shell and IDLE
29 Python Shell
30 Python Editor IDLE
31 Downloadable Cheat Sheet for IDLE Keyboard Shortcuts
32 How to Use Whitespace in Python
33 How to Write and Use Comments in Python
Exploring Python Modules
34 What are Python Modules and How to Use Them
35 How to Import Modules in Python
36 How to Use the dir Method to List Module Attributes
37 How to Use the help Method to Get Module Documentation
38 How to Assign Module Aliases in Python
Controlling the Flow of Python Programs
39 How to Use If Statements in Python
40 Resource if statement
41 How to Use Elif Keyword for Multiple Conditions
42 Resource Elif Statement
43 How to Use If Else Statements for Alternative Actions
44 Resource else statement
45 How to Use AND Operator for Compound Conditions
46 Resource IfAnd Logic
47 OR Condition Within IF Statement
48 Resource IfOR Logic
49 How to Use While Loops for Repeated Execution
50 Resource While Loops
51 How to Use break Keyword to Exit a Loop
52 Resource BREAK Keyword
53 How to Use continue Keyword to Skip an Iteration
54 Resource CONTINUE Keyword
55 How to Use For Loops for Iterating over Sequences
56 Resource For Loops
57 How to Loop through String Values in Python
58 Resource String Looping
59 How to Use Range Function for Generating Numbers in For Loops
60 Resource Range Function
61 How to Use For Loop Else Statement for Handling No Breaks
62 Resource FOR Loop ELSE Statement
Project 1 Magic Questions Game
63 Project 1 Preview
64 Project 1 Instruction Sheet
65 Project 1 Solution Step 1 Setting Up The Magic Question Responses
66 Project 1 Solution Step 2 Capturing Of User Input Respond
67 Project 1 Solution Step 3 Exiting The Application
68 Resource PROJECT1MAGIC QUESTION COMPLETE CODING
Handling Files in Python
69 How to Open and Close Text Files in Python
70 Resource revenuedatatxt
71 Reading Text Files Using Python
72 How to Use a Loop to Read the Entire Content of a Text File
73 Writing To A Text File Within Python
74 How to Create a New Text File in Python
Project 2 Manipulating csv Files with Python
75 What You Will Learn and Do in Project 2
76 Project 2 Instructions and Requirements
77 Resource File empdetails
78 Project 2 Solution Step 1 Importing the csv Module
79 Project 2 Solution Step 2 Opening Reading csv Files
80 Downloadable Reference csvReader Codes
81 Project 2 Solution Step 3 Displaying csv File Data
82 Project 2 Solution Step 4 Creating and Writing to csv Files
83 Resource Completed Codes csvWriter
84 Project 2 Bonus Solution Filtering csv File Data
Working with the OS Module in Python Lectures
85 How to Import the OS Module in Python
86 How to Get the Current Working Directory with the OS getdir Function
87 How to List the Files in a Directory with the OS listdir Function
88 How to Change the Working Directory with the OS chdir Function
89 How to Create a New Directory with the OS makedirs Function
90 How to Check if a File Exists with the isfile Function
91 How to Delete a File with Python
Working With Python Functions
92 Creating a Function in Python
93 Resource Creation Calling Function
94 Calling a Function in Python
95 Passing Arguments to a Python Function
96 Resource Passing Arguments
97 Naming Arguments in a Python Function
98 Resource Named Function
99 Default Arguments in a Python Function
100 Resource Passing DEFAULT Argument
101 Variable Scope of a Python Function
102 Resource Scope Of A Variable
103 Return Keyword in a Python Function
104 Resource return keyword
ObjectOriented Programming OOP in Python
105 How to Define Classes in Python
106 Working with Class Attributes in Python
107 Using the init Method in Python Classes
108 Understanding the self Parameter in Python Classes
109 Creating and Calling Class Methods in Python
110 Python Class Return
111 Implementing Private Properties in Python
112 Defining Private Functions in Python Classes
113 How to Delete Objects in Python
114 Object Inheritance Introduction
115 Resource userpy
116 Python Class Inheritance
117 Overriding Class Inheritance
118 ResourceInheritanceFinal Codes
How to Handle Errors in Python Like a Pro
119 What is Error Handling and Why You Need It
120 Try Statement
121 Try Specific Error Detection
Project 3 WizardryInquiries Game
122 Project 3 Overview
123 Resources Project 3
124 Project 3 Solution Step 1 Setting Up The Class
125 Project 3 Solution Step 2 Implementing The init Method
126 Project 3 Solution Step 3 Implement the Game Functionality
127 Project 3 Solution Step 4 Implementation Of Game Functionality Continued
128 Project 3 Solution Step 5 Write Questions to csv File
129 Project 3 Solution Step 6 Final Run
How to Format Strings in Python
130 What are Formatted Strings and Why Use Them
131 Inserting String Variables into Formatted Strings with s
132 Adding Numeric Values to Formatted Strings with d
133 Using Multiple Variables in a Single Formatted String
134 Formatting Strings with Collection Types like Lists and Dictionaries
Resolve the captcha to access the links!