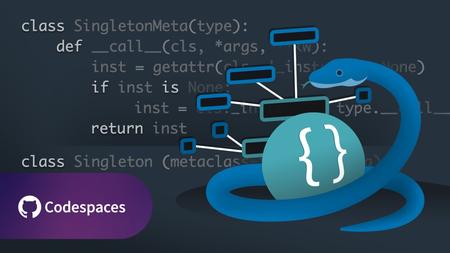
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 1h 21m | 211 MB
In this advanced course, Miki Tebeka, the CEO at 353Solutions, guides you through object-oriented programming features in Python. Learn about attribute access and how to use properties, name mangling, descriptors, and more. Explore using class methods, static methods, mixin classes, and abstract base classes. Dive into sequences, mappings, numbers, and callable types. Find out what metaclasses are and how you can use them. Plus, go over class creation utilities that you’ll need to use. Each chapter comes with a hands-on challenge to practice and reinforce what you learn.
The best way to learn a language is to use it in practice. That’s why this course is integrated with GitHub Codespaces, an instant cloud developer environment that offers all the functionality of your favorite IDE without the need for any local machine setup. With GitHub Codespaces, you can get hands-on practice from any machine, at any time—all while using a tool that you’ll likely encounter in the workplace. Check out the “Using GitHub Codespaces with this course” video to learn how to get started.
Table of Contents
Introduction
1 Advanced object oriented programming (OOP)
2 What you should know
3 Using Codespaces
Attribute Access
4 Deconstructing attribute access
5 Using properties
6 Programmatic attribute access
7 Reducing memory with __slots__
8 Name mangling
9 Descriptors
10 Challenge Colors
11 Solution Colors
Methods
12 Using class methods
13 Using static methods
14 Mixin classes
15 Abstract base classes
16 Defining interfaces with typing.Protocol
17 Challenge Event from JSON
18 Solution Event from JSON
Special Methods
19 String representations
20 Sequences
21 Mappings
22 Numbers
23 Callable
24 Challenge Database-backed dictionary
25 Solution Database-backed dictionary
Metaclasses
26 What are metaclasses
27 Class creation
28 Instance creation
29 Attribute lookup
30 Challenge Singleton
31 Solution Singleton
Class Creation Utilities
32 Class decorators
33 namedtuple()
34 dataclass()
35 Freezing
36 Challenge VM class
37 Solution VM class
Conclusion
38 Next steps
Resolve the captcha to access the links!